Extending JavaScript with macros
What's a macro you say
A macro in computer science is a rule or pattern that specifies how a certain input sequence should be mapped to a replacement output sequence according to a defined procedure.
Translation...
Define our own syntax & extensions to JavaScript
Sweet.js
A macro
The rules and the ouput
macro macroName {
rule {
// The sequence of characters to match
} => {
// The output i.e. valid JavaScript
}
}
Ruby style functions
macro def {
rule {
$fnName:ident
$body ...
end
} => {
function $fnName() {
$body ...
}
}
}
See live demo
Tripple dot ...
Match repeated patterns
macro w {
rule { ($item ...) } => {
[$item (,) ...]
}
}
var arr = w('foo' 1 2 'bar');
// arr = ['foo', 1, 2, 'bar'];
See live demo
Match expressions
By surrounding our pattern in $()
macro hash {
rule { ( $($item => $val) (,) ...) } => {
{
$($item: $val) (,) ...
}
}
}
var obj = hash(x => 1, foo => 'bar');
See live demo
Pattern classes
You can restrict what a macro can match
macro type {
rule { $i:lit } => { $i + 'lit' }
rule { $i:ident } => { $i + 'ident' }
rule { $i:expr } => { $i + 'expr' }
}
type 3; // Literal
type bar; // Identifier
type (1 + 2); // Expression
See live demo
Just the beginning...
- Multiple rules
- Case macros
- Infix rules
- Avoid recursion using let
Infix rules
Do lookbehinds for more complex macros
Fat arrow function macro[1,2,3].map(x => x * x);
Personal extensions
Think of macros as servants in your code
Further reading
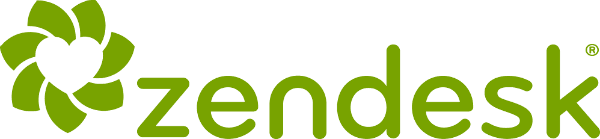